



Anyway, let us explain how a search engine
works in general. Typically, a search engine works by sending out a 'spider' to fetch as many
documents as possible. Then another program, called an indexer, reads these
documents and creates an index based on the
words contained in each document. Each search engine uses a proprietary algorithm to create its indices such that,
ideally, only relevant results are returned for each query.
In the following tutorial we are going to create
a PHP search engine with a MySQL database. We are building our search engine on
PHP 5.2.3. PHP 5.2.3 includes the latest additions such as embedded
SQLite database, support for the MySQL 4.1 client library with the introduction
of the mysqli extension, etc.
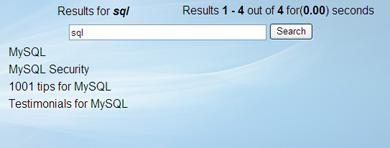
PHP Search Engine
Why should we use MySQL? Well, this pretty
gem will help us organize, fetch and store our data in a quite simple way, so
that we will not need to create our own spider and indexer. MySQL would do the
hard work for us!
Note: For information on how to install and
configure MySQL on Windows you can refer to Gareth Downes-Powell's article "Installing MySQL on a Windows PC".
For installing PHP 5.x.x on Windows you might
want to refer to Allan Kent's Article "Installing PHP 5 under Windows".
Ok, assuming that you have already installed
PHP, MySQL with the appropriate server platform of your choice, IIS or Apache,
you have a fully operational web server. Now, let's get into the details.
For the search engine we are going to build
in this tutorial, I chose to use a table with 3 columns named ID, title, and article, where I have stored the information the user
will be searching for. You can enter some data to your table or even make your
own table. The ID field is the primary key for the table, while title and article are fields in which we plan
to search. To make it easier for you, I`ve prepared a
query to create the table. You just need to copy and paste the following code into the MySQL interface in a database. In
order to do that, access your PHPMyAdmin from your favourite browser, then
select your database and table respectively. There you should notice a tab
named "SQL". You have to paste the following code there:
CREATE TABLE `news` (
`id` tinyint(4) NOT NULL auto_increment,
`title` varchar(200) NOT NULL default '',
`article` text NOT NULL,
UNIQUE KEY `id` (`id`),
FULLTEXT KEY `title` (`title`,`article`)
)
ENGINE=MyISAM AUTO_INCREMENT=1 DEFAULT CHARSET=latin1 AUTO_INCREMENT=1;
Under the "Browse" tab you can see the
entries in your table. In order to insert new entries go to "Insert" tab and
add new values to the fields Title and Article. Surely, you can extend your
table and add additional fields, or change the type of the current fields, in a
whole to change the entire table for your own convenience.
How are we going to proceed? We will start creating
the search form, then setting up our connection to the database, go through the
search function itself, and finally test our search engine. In addition, we are
going to design additional features such as paging and displaying the search
time.
Beginning of the search page…
Firstly let's create our search page with a
form where site visitors will enter keywords to search content. Let's open our favourite
web editor and create a new page. Then insert a new form called searchbox and a
button with value Search. Finally save the page as Search.php. You may want to
use the POST method instead of the GET method for your form since it is more
secure. For this tutorial I chose using the GET method since this allows me to check if the search engine is working properly.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0
Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html;
charset=utf-8" />
<title>Untitled Document</title>
</head>
<body>
<h1>Search Engine with PHP</h1>
<form method="get" action=" ">
<input type="text" name="search_term"
title="Search…" value="Search...">
<input type="submit" name="search" title="Search
Now!
"value="Search" class="searchbutton" />
</form>
</body>
</html>
As you can see this is just simple code for a form with a text field and a
button.



