Free! - How to build a PHP Search Engine
Introduction or a tale of how easy a search engine could work
Five years ago your site may have had five or six pages. However, over the time it has grown and one day you wake up with 2 GB of content, more than a thousand links and no way to sort them by relevance. You need a way for you and your visitors to get information about your services and products faster. A search engine could help you and your site visitors find that precious piece of information on your extensively growing site.




Displaying the results
Now let's display the results. We are going
to use a usual loop where we have saved the first results via the $results
variable. We are arranging everything nicely in tables. That way our results
will be separated and ordered. If no results are found, the page will display
the following message:
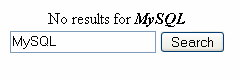
However, if results are found, the page
should look like this:
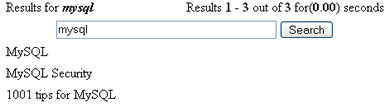
OK, so back to Search.php where we have
inserted our form. Modify the code as follows:
<?PHP
if($results != 0)
{
?>
<table border="0" cellspacing="2"
cellpadding="2">
<tr>
<td width="47%">Results for <?PHP
echo "<i><b><font color=#000000>".$search_term."</font></b></i>
"; ?></td>
<td width="53%" align="right" height="22">Results
<b>
<?PHP echo ($first_pos+1)." - ";
if(($RESULTS_LIMIT + $first_pos) <
$results) echo ($RESULTS_LIMIT + $first_pos);
else echo $results ; ?>
</b>
out of <b><?PHP echo $results;
?></b>
for(<b><?PHP echo sprintf("%01.2f",
$time_search); ?></b>)
seconds </td>
</tr>
<tr>
<form action="" method="GET">
<td colspan="2" align="center">
<input name="search_term" type="text" value="<?PHP echo $search_term;
?>" size="40">
<input name="search_button" type="submit"
value="Search"> </td>
</form>
</tr>
<?PHP
while($row =
mysql_fetch_array($sql_result_query))
{
?>
<tr align="left">
<td colspan="2"><?PHP echo
$row['title']; ?></td>
</tr>
<?PHP
}
?>
</table>
<?PHP
}
elseif($sql_query)
{
?>
<table border="0" cellspacing="2"
cellpadding="0">
<tr>
<td align="center">No results
for <?PHP echo "<i><b><font color=#000000>".$search_term."</font></b></i>
"; ?></td>
</tr>
<tr>
<form action="" method="GET">
<td colspan="2" align="center">
<input name="search_term" type="text"
value="<?PHP echo $search_term; ?>">
<input name="search_button" type="submit"
value="Search">
</td>
</form>
</tr>
</table>
<?PHP
}
?>
Sorting into pages
Our final step is to show the number of result
pages. We are calculating how many results the SQL query has returned. We start
by calculating the first position in order to place it in relevance. If the
maximum number of results is exceeded the algorithm creates a forward button for the user to switch to the next
page. We already have defined the maximum number per page via $RESULTS_LIMIT. If
the user goes to the second page of the results he can go back and check
previous results with a back button. Well, these so called buttons are not
really buttons – they are simple ">>" and "<<" signs. Of course
feel free to add your own buttons, or even some flash images.
I am also using a PHP function stripslashes
in order to escape some quotes. Why? I
use it to clean the results from the quotes in the MySQL query. That way the
algorithm will not fail if any quotes are inserted.
We can do all this with the following code:
<?PHP
if($first_pos > 0)
{
$back=$first_pos-$RESULTS_LIMIT;
if($back < 0)
{
$back = 0;
}
echo "<a href='search.php?search_term=".stripslashes($search_term)."&first_pos=$back'
></a>";
}
if($results>$RESULTS_LIMIT)
{
$sites=intval($results/$RESULTS_LIMIT);
if($results%$RESULTS_LIMIT)
{
$sites++;
}
}
for ($i=1;$i<=$sites;$i++)
{
$fwd=($i-1)*$RESULTS_LIMIT;
if($fwd == $first_pos)
{
echo "<a href='search.php?search_term=".stripslashes($search_term)."&first_pos=$fwd
'><b>$i</b></a> | ";
}
else
{
echo "<a href='search.php?search_term=".stripslashes($search_term)."&first_pos=$fwd
'>$i</a> | ";
}
}
if(isset($first_pos) && $first_pos <
$results-$RESULTS_LIMIT)
{
$fwd=$first_pos+$RESULTS_LIMIT;
echo "<a href='search.php?search_term=".stripslashes($search_term)."&first_pos=$fwd
' > >></a>";
$fwd=$results-$RESULTS_LIMIT;
}
?>
</td>
</tr>
</table>
This is the final piece of code in this
article.
The next code block contains the entire
code for the search engine. I've inserted comments so that you can track the
process:
<?PHP
function getmicrotime()
{
list($usec, $sec) = explode(" ", microtime());
return ((float)$usec + (float)$sec);
}
//initializing connection to the database
$connection_string = dirname(__FILE__) . "/connectionstring.php";
require_once($connection_string);
//selecting table
mysql_select_db("test") or die ( 'Unable to select database.' );
//max number of results on the page
$RESULTS_LIMIT=10;
if(isset($_GET['search_term']) && isset($_GET['search_button']))
{
$search_term = $_GET['search_term'];
if(!isset($first_pos))
{
$first_pos = "0";
}
$start_search = getmicrotime();
//initializing MySQL Quary
$sql_query = mysql_query("SELECT * FROM news WHERE
MATCH(title,article) AGAINST('$search_term')");
//additional check. Insurance method to re-search the
database again in case of too many matches (too many matches cause returning of
0 results)
if($results = mysql_num_rows($sql_query) != 0)
{
$sql = "SELECT * FROM news WHERE MATCH(title,article) AGAINST('$search_term')
LIMIT $first_pos, $RESULTS_LIMIT";
$sql_result_query = mysql_query($sql);
}
else
{
$sql = "SELECT * FROM news WHERE (title LIKE '%".mysql_real_escape_string($search_term)."%'
OR article LIKE '%".$search_term."%') ";
$sql_query = mysql_query($sql);
$results = mysql_num_rows($sql_query);
$sql_result_query = mysql_query("SELECT * FROM news WHERE (title LIKE '%".$search_term."%'
OR article LIKE '%".$search_term."%') LIMIT $first_pos, $RESULTS_LIMIT ");
}
$stop_search = getmicrotime();
//calculating the search time
$time_search = ($stop_search - $start_search);
}
?>
<?PHP
if($results != 0)
{
?>
<!-- Displaying of the results -->
<table border="0" cellspacing="2" cellpadding="2">
<tr>
<td width="47%">Results for <?PHP echo "<i><b><font
color=#000000>".$search_term."</font></b></i> ";
?></td>
<td width="53%" align="right" height="22">Results
<b>
<?PHP echo ($first_pos+1)." - ";
if(($RESULTS_LIMIT + $first_pos) < $results)
echo ($RESULTS_LIMIT + $first_pos);
else echo $results ; ?>
</b>
out of <b><?PHP echo $results;
?></b>
for(<b><?PHP echo sprintf("%01.2f",
$time_search); ?></b>)
seconds </td>
</tr>
<tr>
<form action="" method="GET">
<td colspan="2" align="center"> <input
name="search_term" type="text" value="<?PHP echo $search_term; ?>" size="40">
<input name="search_button" type="submit"
value="Search"> </td>
</form>
</tr>
<?PHP
while($row = mysql_fetch_array($sql_result_query))
{
?>
<tr align="left">
<td colspan="2"><?PHP echo
$row['title']; ?></td>
</tr>
<?PHP
}
?>
</table>
<?PHP
}
//if nothing is found then displays a form and a message that there are nor
results for the specified term
elseif($sql_query)
{
?>
<table border="0" cellspacing="2" cellpadding="0">
<tr>
<td align="center">No results
for <?PHP echo "<i><b><font color=#000000>".$search_term."</font></b></i>
"; ?></td>
</tr>
<tr>
<form action="" method="GET">
<td colspan="2" align="center">
<input name="search_term"
type="text" value="<?PHP echo $search_term; ?>">
<input
name="search_button" type="submit" value="Search">
</td>
</form>
</tr>
</table>
<?PHP
}
?>
<table width="300" border="0" cellspacing="0" cellpadding="0">
<?php
if (!isset($_GET['search_term'])) { ?>
<tr>
<form action="" method="GET">
<td colspan="2" align="center">
<input
name="search_term" type="text" value="<?PHP echo $search_term; ?>">
<input
name="search_button" type="submit" value="Search">
</td>
</form>
</tr>
<?php
}
?>
<tr>
<td align="right">
<?PHP
//displaying the number of pages where the results are sittuated
if($first_pos > 0)
{
$back=$first_pos-$RESULTS_LIMIT;
if($back < 0)
{
$back = 0;
}
echo "<a href='search.php?search_term=".stripslashes($search_term)."&first_pos=$back'
></a>";
}
if($results>$RESULTS_LIMIT)
{
$sites=intval($results/$RESULTS_LIMIT);
if($results%$RESULTS_LIMIT)
{
$sites++;
}
}
for ($i=1;$i<=$sites;$i++)
{
$fwd=($i-1)*$RESULTS_LIMIT;
if($fwd == $first_pos)
{
echo "<a href='search.php?search_term=".stripslashes($search_term)."&first_pos=$fwd
'><b>$i</b></a> | ";
}
else
{
echo "<a href='search.php?search_term=".stripslashes($search_term)."&first_pos=$fwd
'>$i</a> | ";
}
}
if(isset($first_pos) && $first_pos < $results-$RESULTS_LIMIT)
{
$fwd=$first_pos+$RESULTS_LIMIT;
echo "<a href='search.php?search_term=".stripslashes($search_term)."&first_pos=$fwd
' > >></a>";
$fwd=$results-$RESULTS_LIMIT;
}
?>
</td>
</tr>
</table>
Do not forget to upload the files
connectionstring.php and Search.php in the same directory on your server.
Preview your page and test it. Just open it
in your favourite web browser and type in some words. Make sure that your table
is filled with some of these words otherwise it will return you that there are
no results found.




Kiril Iliev started his career as a PR manager for a Bulgarian game software company and later on started working for Dynamic Zones - the company behind the zones network.
He has written a lot of articles for one of the biggest computer magazines in Bulgaria - PC Mania.
He works on various projects including PHP, CSS, and ASP scripting and is support specialist at DMXzone.com.
As of April, 2008 Kiril is acting as Research and Development Manager, researching the trends in the new technologies, especially Silverlight and LINQ implementation
See All Postings From Kiril Iliev >>
Reviews
PHP search engine
There seems to be a problem with this search engine. In IE when pressing enter as oppose to search button, nothing displays. Is there any way to fix it?
Paging issues
Thanks alot for the guide, great job! Although the paging part doesn't work for me. When i select the next page i just find myself on an empty page. I haven't changed anything but the things i have to change. search.php?search_term=mysql&search_button=Search works but when i turn to the next page : search.php?search_term=mysql&first_pos=10 the page is empty. Any way to fix this problem? I have followed many search engine guides and i find this problem on every single one of them, it's getting really enoying. Maybe it's my fault some how, help would be really nice :)
ERROR!
hello! i followed the steps that you've indicated in this *tutorial about making a search engine in php. I do all the steps including the database but when i run it, theres an error saying this Parse error: syntax error, unexpected T_VARIABLE in C:\Documents and Settings\Personal\Desktop\madeL\connectionstring.php on line 15
what do you think is wrong with what ive done? pls help me coz i needed it for my thesis. plssss...
NOTE: i used POST method instead of GET method. i also used DREAMWEAVER 2004 and WAMMP. plss response. thank yoU..
See all 8 Reviews You must me logged in to write a review.